- Python
- Java
- Javascript / Jquery
- C, C# & C++
- SQL
- PHP
- Go
- Ajax
- Visual Basic (ms office)
- Angular
- Laravel
- .net & asp.net
- Flex, Flash
- Linux
- Swift
- Rust
- Matlab
- Django
- Ruby On Rails
- MEAN Stack
- MERN Stack
- MEVN Stack
- LAMP Stack
- LEMP Stack
- LAPP Stack
- LEAP Stack
- LLMP Stack
- XAMPP Stack
- WAMP Stack
- WIMP Stack
- MAMP Stack
- Flutter Stack
- Serverless Stack
- Misc & Others
- Browse All Categories
Master Python Programming: A Comprehensive Guide for Beginners and Experts
Python is a very popular and widely used programming language that mainly focuses on simplicity and readability. Its popularity has grown over all these years because it has an uncomplicated syntax and maintains a clean style of coding, along with various helpful tools. Python programming language was developed by a Dutch programmer Guido van Rossum, as a successor to the ABC programming language. He developed Python with the intention of creating a user-friendly and comprehensible language. Since then, a community of developers has contributed to its improvement and overall development. Python 3 is the current version of Python which was released in 2008. Introduced new features while maintaining backward compatibility with the previous versions. Python is a versatile programming language and whether you are a novice or an experienced programmer you need to understand Python's strengths and capabilities which is very essential in today's tech-driven world.
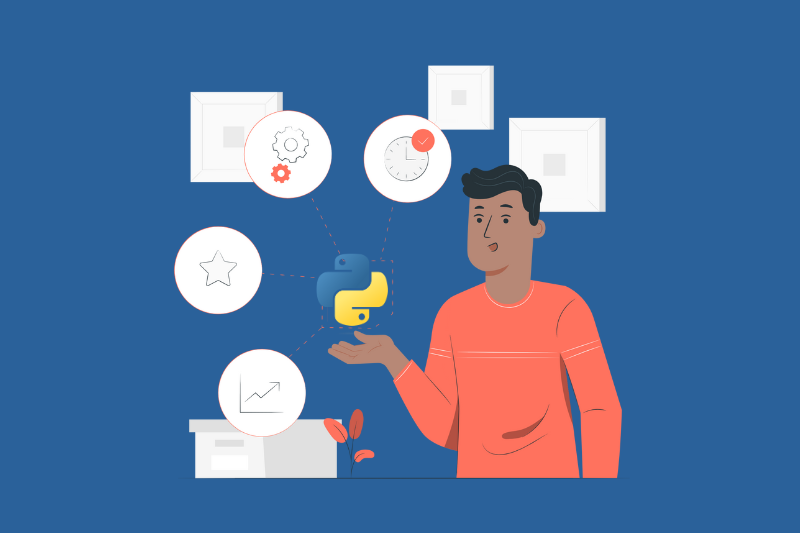
Features and Benefits of Python
Python offers numerous features that contribute to its popularity among developers. The following are the main features of the Python programming language:
- Clean syntax and whitespace indentation for readable code
- Cross-platform compatibility for diverse operating systems
- Extensive standard library for time-saving modules and functions
- A vast ecosystem of external packages for expanded capabilities
- Simplified development process with quick prototyping
- Active and supportive community for valuable resources and collaboration
Getting Started with Python
Before jumping into the world of Python programming, you have to first set up your development environment. You need to follow these steps in order to get started:
Installing Python
Download Python from the official website of Python(https://www.python.org/downloads/) and. Once downloaded, locate the file and run the installer. This will install Python on your machine. Make sure that you select the option to add Python to the system PATH during the installation process.
Setting up the Development Environment
After successful installation of Python, you can choose an integrated development environment (IDE) of your choice such as PyCharm, Visual Studio Code, and Sublime Text. Alternatively, you can also use a text editor like Notepad++ to write your Python code. Choose according to your preference and then install it.
Running Your First Python Program
After setting up the development environment, open your chosen IDE or text editor and create a new Python file. In order to print"Hello, Python!" type the following code on the console:
Save the file with a .py extension and run it. You should see the output Hello, Python! displayed in the console, indicating that Python is correctly installed and configured.
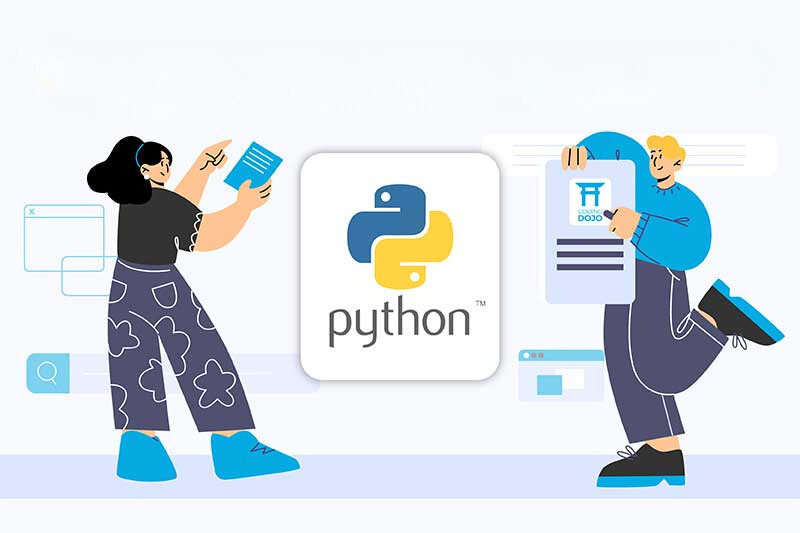
Python Syntax and Data Types
If you aim at writing effective Python code, it’s quite essential that you first understand its syntax as well as its various data types. This knowledge forms the foundation for creating programs and manipulating data. Let's explore some of the fundamental aspects of Python syntax and data types.
Variables and Constants
In Python, you can assign values to variables using a simple assignment operator (=). A variable can store different data types such as numbers, strings, lists, and even complex objects while constants don't change at all. They are the values that remain unchanged throughout the program’s execution.
Operators and Expressions
There are a lot of operators in Python which are arithmetic, comparison, assignment, logical, and more. These operators allow you to perform calculations, make comparisons, and modify variable values based on specific conditions. The expressions are made with a combination of variables, constants, and operators and are used to perform computations and generate results.
Control Flow Statements
Control flow statements consist of if-else, for loops, while loops, and conditional statements in Python. They enable you to control the flow of execution in your Python code. They allow you to make decisions, iterate over collections, and execute specific blocks of code based on certain conditions.
Data Structures in Python
Lists, tuples, sets, and dictionaries are the built-in data structures in Python. They help you to organize and manipulate data efficiently. Each data structure serves a specific purpose and offers different methods and operations for data storage and retrieval.
Functions and Modules in Python
Functions and modules play a crucial role in modularizing code and promoting reusability. Let's explore how to create and use functions in Python and understand the concept of modules and libraries.
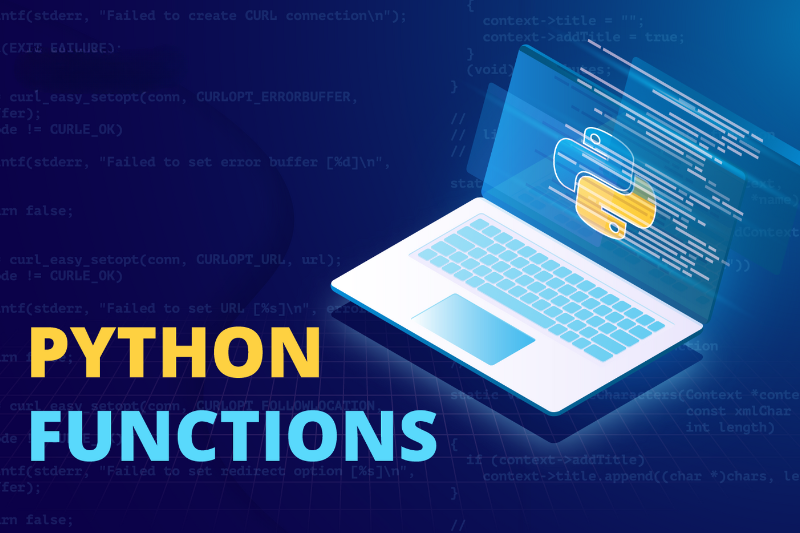
Creating and Using Functions
In order to create a function in Python the def keyword is used with the function name and a set of parentheses You can define parameters inside the parentheses to accept input values. Functions can return values using the return statement. By encapsulating specific blocks of code into functions, you can easily reuse and call them whenever needed.
Understanding Modules and Libraries
In Python modules are various files that contain Python code. These files can be imported and used in other programs. Modules allow you to organize your code into logical units and avoid code duplication. Python's extensive library ecosystem provides a wide range of pre-built modules that you can import and leverage in your projects.
Object-Oriented Programming in Python
Python has support for object-oriented programming which allows you to create objects and manipulate them by adding several attributes. Understanding OOP concepts is essential for building complex and scalable applications. Let's explore the key aspects of OOP in Python.
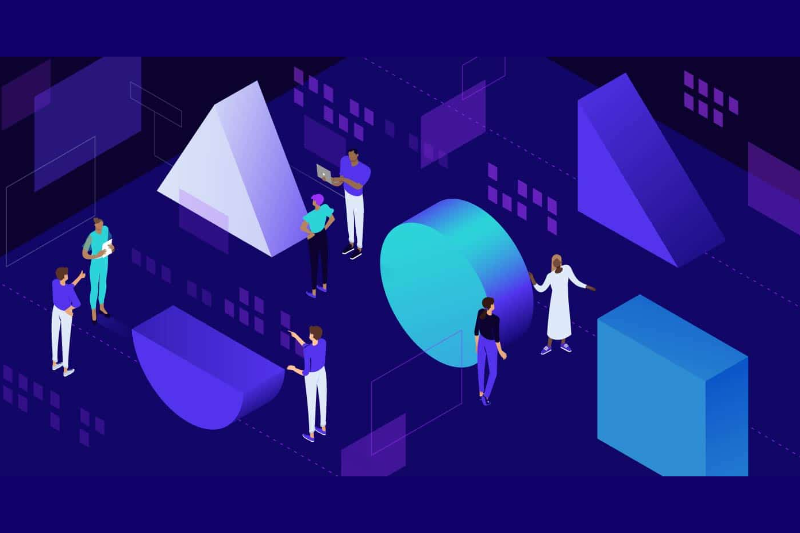
Classes and Objects
In Python, classes are blueprints for creating objects. A class defines the attributes (data) and methods (functions) that objects of that class can possess. Objects are instances of a class, and each object has its own unique data and behavior while following the class's defined structure.
Inheritance and Polymorphism
Inheritance refers to possessing properties of an existing class or base class by a new class or derived class. This enables code reuse and promotes modularity. Polymorphism allows objects of different classes to be treated as objects of a common superclass. This helps to simplify the code design and make it more flexible.
Encapsulation and Abstraction
Encapsulation refers to the bundling of data and methods within a class, preventing direct access to the internal state of an object. It ensures data integrity and promotes modular code design. Abstraction involves representing complex entities by their essential characteristics, hiding unnecessary details, and exposing only the relevant information.
File Handling in Python
File handling refers to reading and writing data to and from files. It is in fact an important part of many applications. You can easily handle files with different file formats in Python and for this, it offers many efficient methods.
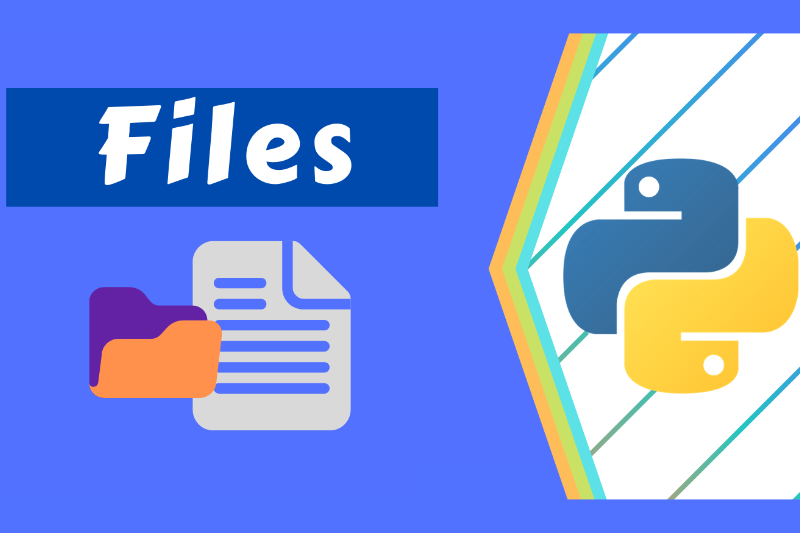
Reading and Writing Files
The open() function in Python is used to read the contents of a file. There are three modes for this which are read ('r'), write ('w'), or append ('a'). After reading or writing, it's crucial to close the file using the close() method to free up system resources.
Working with CSV and JSON Files
The process of reading and writing CSV (Comma-Separated Values) files is made very easy by Python's csv module. It is normally used for data storage and exchange. Another module called the JSON module, allows you to work with JSON (JavaScript Object Notation) files, which are used for data serialization and communication between systems.
Python Libraries and Frameworks
Python's extensive library ecosystem offers a wealth of third-party libraries and frameworks that expand its capabilities for various domains and use cases. Let's explore some popular Python libraries and two widely used web frameworks.
Overview of Popular Python Libraries and Frameworks
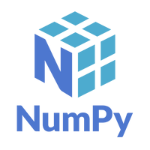
NumPy
A fundamental library for scientific computing and numerical operations, providing powerful data structures and functions for efficient computation.
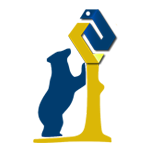
Pandas
A versatile library for data manipulation and analysis, offering easy-to-use data structures and data analysis tools.
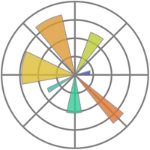
Matplotlib
A plotting library that lets you create various types of static, animated, and interactive visualizations in Python.
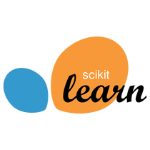
Scikit-Learn
A machine learning library that offers a wide range of tools in order to conduct machine learning tasks such as classification, regression, clustering, and more.
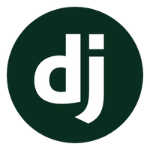
Django
A high-level web framework that follows the model-view-controller (MVC) architectural pattern. Django simplifies web development by providing robust tools and conventions for building secure and scalable web applications.
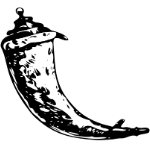
Flask
A lightweight and flexible web framework that follows a microservices approach. Flask is highly customizable and allows developers to choose components according to their project's specific requirements.
Python for Data Science and Machine Learning
Python has gained huge popularity in the domain of data science and machine learning communities. The credit for this goes to its extensive libraries and frameworks. Let's explore some essential tools for data science and machine learning in Python.
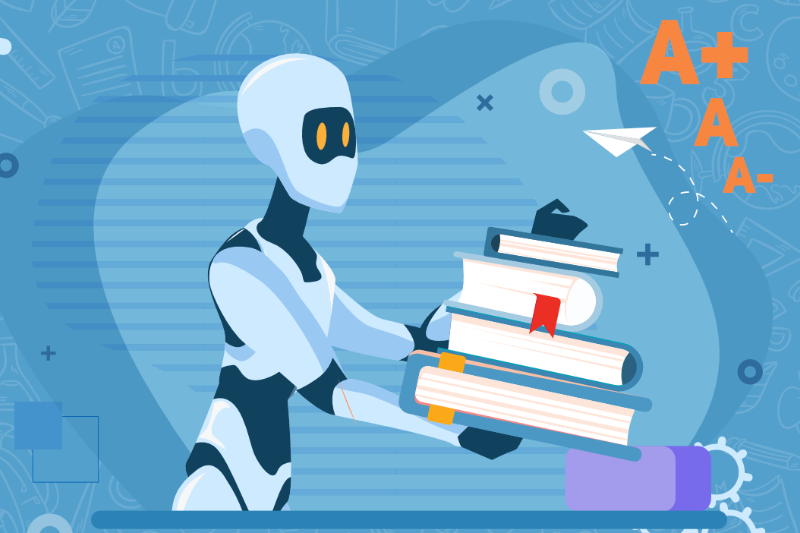
-
1
NumPy and Pandas for Data Manipulation
NumPy and Pandas are powerful libraries for data manipulation and analysis.
NumPy provides multidimensional arrays and a collection of mathematical functions for efficient numerical computations. Pandas is built on top of NumPy. It offers high-level data structures and functions which makes it easier to work with structured data. -
2
Scikit-Learn For Machine Learning
Scikit-Learn is a comprehensive machine-learning library that offers a wide range of algorithms and tools for classification, regression, clustering, and model evaluation. It makes the process of building machine learning models quite easy. It also supports integration with libraries like NumPy and Pandas.
-
3
Visualizing Data With Matplotlib And Seaborn
Matplotlib and Seaborn are popular libraries for data visualization in Python. Matplotlib allows you to create a wide range of static, animated, and interactive plots, while Seaborn provides a higher-level interface for creating aesthetically pleasing statistical visualizations.
Web Development with Python
Python is quite popular in the field of web development. It has powerful web frameworks and libraries that make the process of web development easier Python provides the following frameworks for web development.
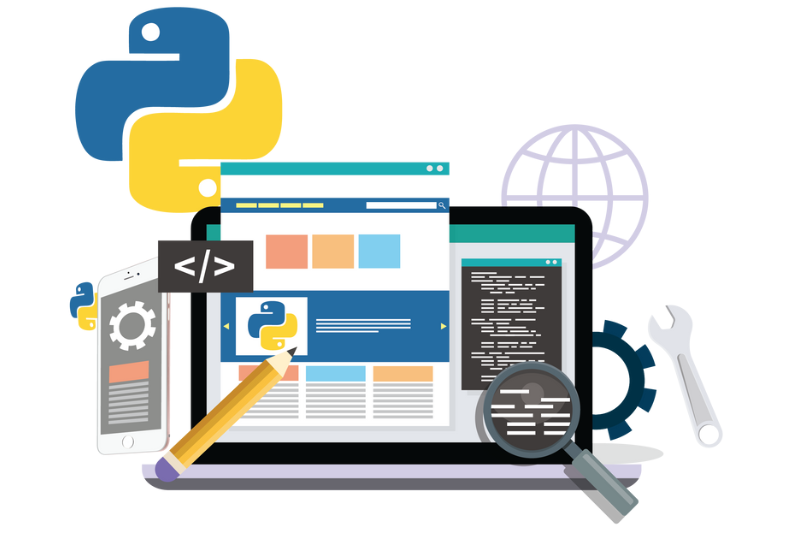
Building Web Applications with Django
Django is a robust web framework that follows the model-view-controller (MVC) architectural pattern. It provides a high-level API and built-in features for handling routing, database interactions, user authentication, and more. Django's batteries-included approach accelerates web application development.
Creating APIs with Flask
Flask is a lightweight web framework and is perfect for building RESTful APIs. It provides a simple yet flexible approach to web development that allows developers to design and implement APIs with ease. Flask's simplicity and extensibility make it a popular choice for creating web services and microservices.
Python in the Artificial Intelligence and Automation Space
Python's versatility extends to the fields of artificial intelligence (AI) and automation. Let's explore how Python can be used in these domains.
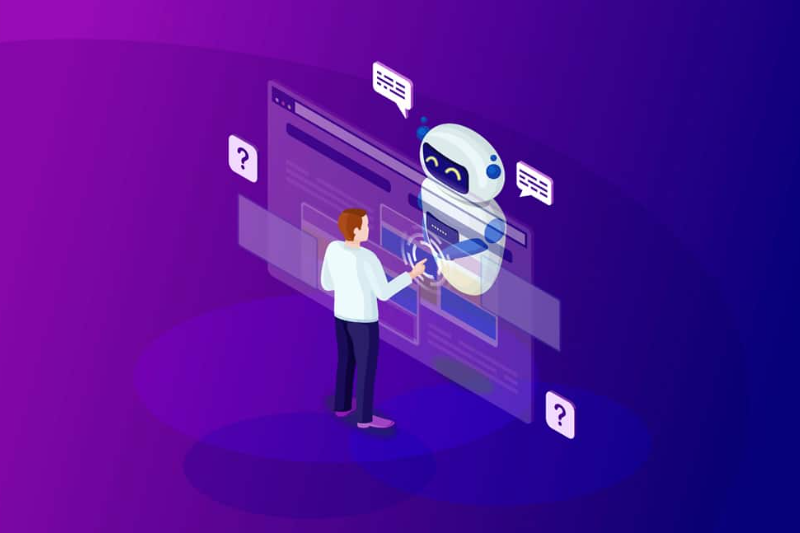
Natural Language Processing with NLTK
Natural Language Processing (NLP) is a subfield of AI. It mainly focuses on understanding and generating human language. The Natural Language Toolkit (NLTK) is another robust library for NLP in Python that provides a set of tools for text preprocessing, part-of-speech tagging, sentiment analysis, and more.
Computer Vision with OpenCV
Computer Vision is a field that deals with processing and analyzing digital images and videos. OpenCV (Open Source Computer Vision) is a widely used library in Python that is dedicated to computer vision tasks. It provides a wide range of functions for tasks like image processing, object detection, and image recognition.
Frequently asked Question
Learn about features from FAQ!
A: Python is a high-level and versatile programming language that is quite simple and readable. It emphasizes code readability and has a large community of developers. It is used in the areas of web development, data analysis, artificial intelligence, and automation.
A: Python is undoubtedly the best programming language for beginners. Python offers an easy-to-read syntax and the code is quite easy to read too. It also has a supportive community and huge learning resources available which makes it a perfect choice for beginners.
A: There are numerous online resources, tutorials, and courses available to learn Python. There are certain websites such as Codecademy, Coursera, and Udemy which offer Python courses both for beginners and advanced learners. Additionally, the official Python website provides comprehensive documentation and tutorials for self-learning.
A: In order to install Python you need to visit the official website of Python at python.org and then download the latest version which is compatible with the OS your machine is running.
A: Python has excellent dedicated frameworks like Django and Flask for web development, which simplify web application development and facilitates the building of robust and scalable websites and web services.
A: NumPy, Pandas, matplotlib, and Scikit-Learn are some of the popular Python libraries for data science. These libraries offer the required tools for data manipulation, analysis, visualization, and machine learning.
A: Yes, Python is extensively used in AI and automation. Libraries like NLTK and OpenCV enable natural language processing and computer vision tasks, respectively, while frameworks like TensorFlow and PyTorch facilitate deep learning and neural network-based AI applications.
A: There are tons of benefits to using Python. It has a clean and readable syntax, has a huge library of frameworks, is cross-platform compatible, and has strong community support. It is best suited for beginners and experienced developers.
A: Python is quite versatile and can be used for multiple purposes. It can be used for web development, data analysis and visualization, machine learning, artificial intelligence, automation, scientific computing, and more. Its extensive library ecosystem makes it suitable for various tasks.
A: Python libraries are pre-written code modules that provide additional functionalities to Python programs. These modules are developed and maintained by the Python community and cover various domains such as data manipulation, scientific computing, web development, machine learning, and more. NumPy, Pandas, matplotlib, and TensorFlow are some of the widely used libraries in Python.
A: Yes, indeed! Python is one of the most popular programming languages for data analysis and machine learning. Libraries like Pandas, NumPy, and Scikit-Learn provide powerful tools for data manipulation, analysis, and machine learning. Python's simplicity and the availability of these libraries make it a popular choice among data scientists and machine learning practitioners.
A: Yes, the Python community encourages contributions from developers of all levels. You can contribute to the Python language as well as to various open-source projects and libraries in the Python ecosystem. Contributing can involve writing code, improving documentation, reporting bugs, or helping others in the community.
Conclusion
To wrap up, Python is a versatile and robust programming language that offers tons of benefits to developers. Its clean syntax, cross-platform compatibility, and an extensive standard library make it a perfect choice for both beginners and seasoned developers. With a vast ecosystem of third-party packages and a supportive community, Python empowers developers to tackle complex tasks efficiently. Python excels in every field, whether it's web development, data analysis, machine learning, or automation, Python's simplicity and rich set of tools make it a top choice for programmers worldwide. Its readability, rapid prototyping capabilities, and strong community support make it a leading programming language in today's technology landscape.